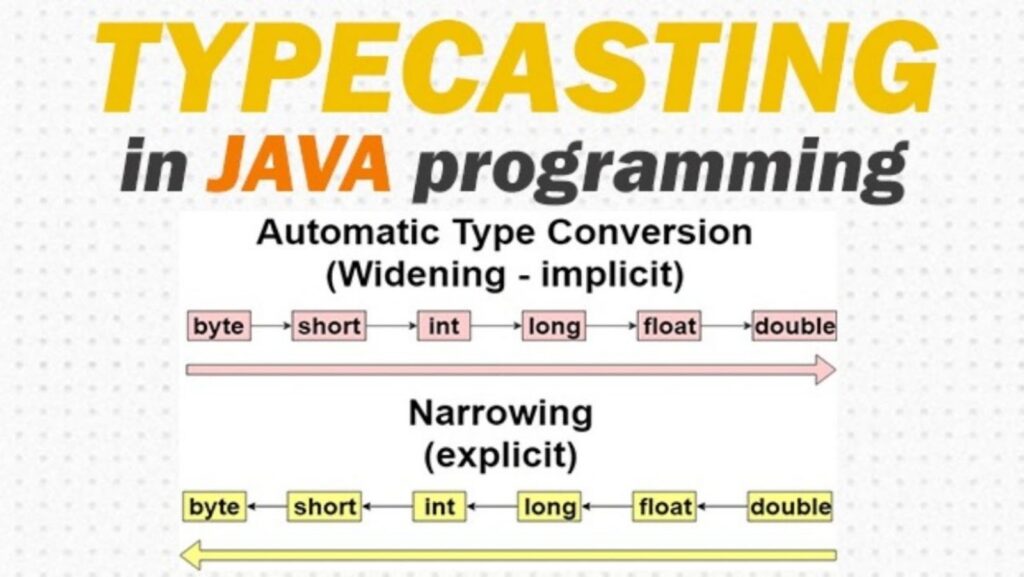
There are four main types of casting in Java:
1. Upcasting
2. Downcasting
3. Widening primitive conversion
4. Narrowing primitive conversion
What is Upcasting in java
Upcasting is the process of converting a child class object into a parent class object. The compiler automatically performs this type of casting. For example, if we have a Parent class and a Child class, and we create an object of type Child, we can assign it to a variable of type Parent:
Child c = new Child();
Parent p = c; // upcasting
What is downcasting in java
Downcasting is the process of converting a parent class object into a child class object. The compiler will not automatically perform this type of casting, so we must explicitly specify that we want to downcast an object:
Parent p = new Child();
Child c = (Child) p; // downcasting
What is Widening primitive conversion in java
Widening primitive conversion is the process of converting a smaller primitive data type into a larger primitive data type. The compiler will automatically perform this type of casting. For example, we can convert a byte value into an int value:
byte b = 1;
int i = b; // widening primitive conversion
What is Narrowing primitive conversion in java
Narrowing primitive conversion is the process of converting a larger primitive data type into a smaller primitive data type. The compiler will not automatically perform this type of casting, so we must explicitly specify that we want to narrow an object:
int i = 1;
byte b = (byte) i; // narrowing primitive conversion
When we try to upcast or downcast an object, the compiler will check to see if the conversion is valid. If the conversion is not valid, the compiler will generate an error.
Benefits of casting
Casting can be useful in the following situations:
When we need to convert a parent class object into a child class object so that we can access the child class methods.
When we need to convert a primitive data type into another primitive data type so that we can perform operations that are not available for the original data type.
Drawbacks of casting
Casting can also be dangerous and can lead to runtime errors. For example, if we try to downcast a Parent object into a Child object, but the Parent object does not actually contain a Child object, we will get a ClassCastException:
Parent p = new Parent();
Child c = (Child) p; // ClassCastException!
This is why it’s always a good idea to check whether an object can be safely cast into another object before actually performing the cast.
Why do we need to cast objects in Java
Casting is a way of converting one type of data into another type of data. In Java, there are two types of casting: primitive conversion and reference conversion.Primitive conversion is the process of converting one primitive data type into another primitive data type. For example, we can convert an int value into a byte value.Reference conversion is the process of converting one reference data type into another reference data type. For example, we can convert a Child object into a Parent object.
Conclusion
Casting is a powerful feature of Java that can be used to convert an object of one type into another type. However, casting can also be dangerous and can lead to runtime errors if not used properly. Always check whether an object can be safely cast into another object before actually performing the cast.